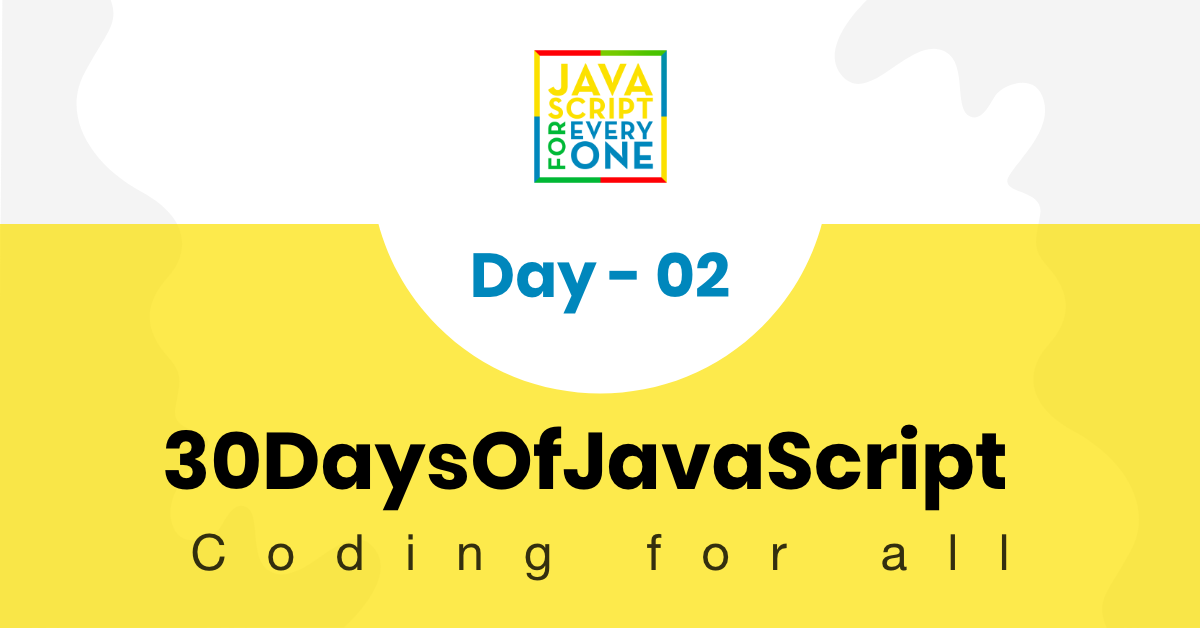
Day 2 Recap of 30DaysofJavaScript
August 4, 2022 • 5 min read
Finished Day 2! Let's recap!
So, how did Day 2 of 30DaysOfJavaScript go?
Well, for starters, I feel it was slightly overhwhelming. This was because of the vast knowledge that was put on me about Strings and String Methods.
Learning about how each String Method worked was tough, but I also know it is essential to know going forward, especially for anything taught may require string manipulation in a future project either in this course or the future.
Click here to follow along.
I am getting a little ahead of myself, so let's go from the top!
Primitive vs Non-Primitive Data Types
On Day 1 I was introduced to the various data types in JavaScript. Day 2 went further into this topic, and introduced the concept of Primitive Data Types and Non-Primitive Data Types.
Primitive Data Types
Primitive Data Types include the following:
- Numbers(integers and floats)
- Strings(data in between ' ', " ", or ` `)
- Booleans(true or false)
- Null(empty/no value
- Undefined(variable with no value)
- Symbol(unique value generated by Symbol constructor
Primitive data types are immutable, or non-modifiable. This means that once you create a primitive data type, you cannot modify it. An example would be like:
let variable = 'Jovin'
//you cannot then modify the string like this:
variable[1] = 'X'
//JavaScript would raise an error
Primitive data types can be compared by values. You can use comparisons such as "==" to check if the values of two variables are equal.
Non-Primitive Data Types
On the other hand, Non-primitive Data Types include the following:
- Objects
- Arrays
Non-primitive data types are mutable, which means the value can be modified. For example, let's take an array called nums:
let nums = [ 1 , 10 , 100 ]
If we were to
nums[1] = 200
If we were to print to the console, the new array would be [1, 200, 100]
With Non-primitive data types, you cannot compare by value like primitive data types, even if they have the same property and values. Rather, they are compared by reference. This means that two objects are only stricty equal to each other if they are referenceing the same object.
let nums = [ 1 , 10 , 100 ]
let numbers = [ 1 , 10 , 100 ]
console.log(nums == numbers) //this would come as false
let nums = [ 1 , 10 , 100 ]
let numbers = nums
console.log(nums == numbers) //this would come as true
Numbers
In JavaScript, the Math Object has a lot of methods to work with number data types. I'll list a few of them here, you can read more about them on Day 2 of 30DaysofJavaScript.
- Math.round() - rounds to nearest number
- Math.floor() - rounds down
- Math.ceil() - rounds up
- Math.random() - generates a random number between 0 and 0.999999
- Math.log(x) - returns the natural log of x
To use the random() method, you have to set it up so it can gernerate numbers >=1
let randomNum = Math.random()
let betweenZeroAndTen = randomNum * 11 // gives number between 0-10.99
let randomNumRoundFloor = Math.floor() // will give number between 0 & 10
Strings
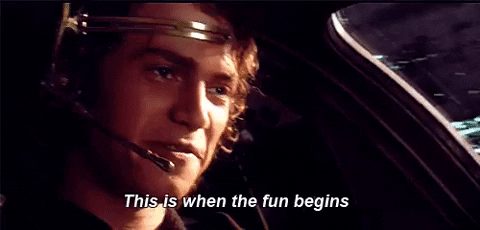
Oh boy this is NOT where the fun began.
Strings... man this nearly broke me. So many methods to learn, so many examples to read.
In all seriousness, none of the string methods were hard to understand, but rather, they were sheer amount of information overwhelmed me at first read.
But before we get into the string methods, let's go over a few things...
String Concatenation
If you connect 2 or more strings, that would be concatnation. You CAN use the addition operator to do so, as shown in the code below:
let fullName = firstName + space + lastName
However, this way as said in the lesson is tedious and error prone. THe other way is to use ES^ template strings, which would be explained at a later time.
Long Literal Strings, Escape Sequences, and Template Strings
If you wanted a string to be long, but cannot fit it on one line, use the backslash(\) at the end of each line. This will let JavaScript know the string will continue on the next line.
const paragraph = "This is an example of how to use a long literal string.\
This way, the string can be used on multiple line. Using the backslash \
character indicated you want the string to recoginze another line as\
being part of the same string."
In JavaScript, as well as other programming languages, you use the \ followed by characters to make an escape sequence. These include:
- \n new line
- \t tab
- \\ back slash
- \' single quote
- \" double quote
Inside ` `, you can inject data in a template string by enclosing the expression with curly brackets {}:
let a = 2
let b = 3
console.log(`The sum of ${a} and ${b} is ${a+b}`)
// this will print out the data for a & b dynamically
String Methods
With the 20 string methods in this section of Day 2, I will share just some of them. If you would like to read about all of them, check out Day 2 of 30DaysOfJavaScript!
- length: returns length of string
- toUpper & toLower: makes the string either all uppercase or lowercase respectively
- substr():slices a given number of characters in string at starting index
- split(): splits a string at a specified place
- includes(): returns ture or false after checking if a substring arguement exists within a string
- indexOf(): given a substring, if subnstring exists in string, returns first position of substring
- concat(): takes substrings and joins them
- repeat(): takes a number and returns a string repeated that many times
And this is the end of my recap of Day 2 of 30DaysofJavaScript. A lot more information from this day, but it was definitely a good recap.
You can check out my GitHub repo, where I stored my code from the stream, which has code examples and comments/notes I made about what I was learning.
Day 3 should be coming out soon! If you want to know when it comes out, subscribe to the blog below! Share if you found my content interesting, and consider buying me some boba with the link down below.
Until next time, wherever, and whenever you are, have an amazing day! Signing off.
If you liked this and would like to support, click the link below to send me a boba!!! 🧋