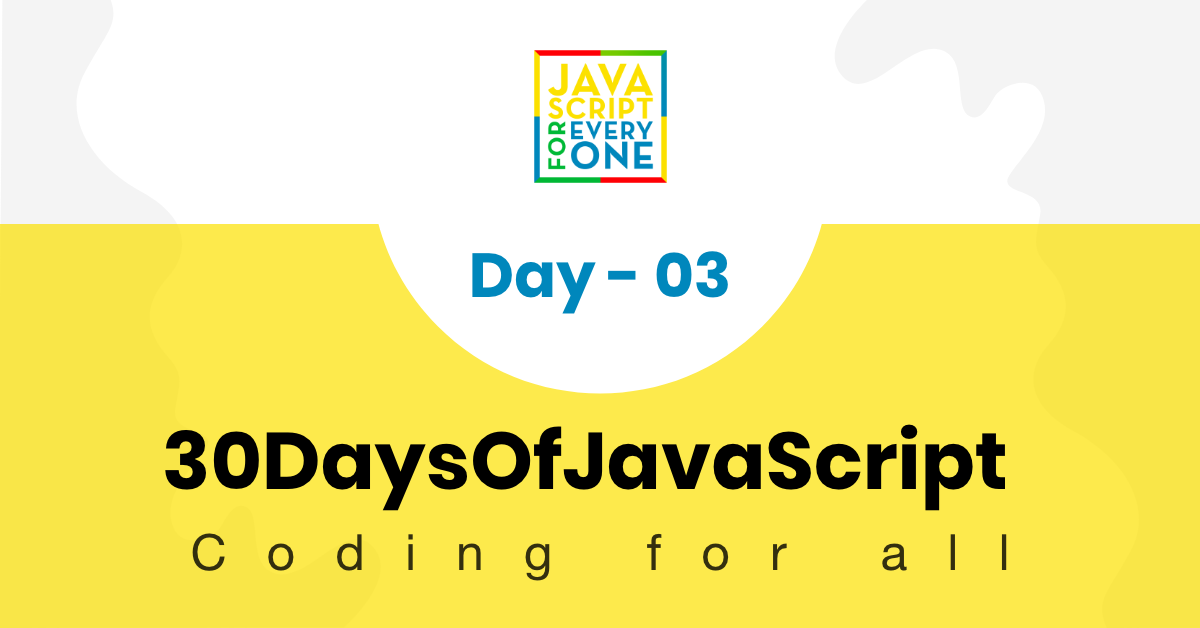
Day 3 Recap of 30DaysofJavaScript
August 10, 2022 • 4 min read
Finished Day 3! Let's recap!
So, what did I learn on Day 3 of 30DaysofJavaScript? Let's see!
The topics taught in Day 3 were Booleans, Undefined, Null, Operators, Window Methods, and Date Objects.
Click here to follow along.
Let's go through each one!
Booleans
So, booleans are a data type that will return as either true or false. You can use booleans when using comparisons, as comparisons while return a boolean value.
Truthy Values
The following values will be true:
- All positive and negative numbers (not zero)
- All strings except an empty string
- Boolean true
Falsy Values
The following values will be true:
- 0
- 0n
- null
- undefined
- Not a Number (NaN)
- Boolean false
- Empty string
Undefined & Null
The undefined and null sections were brief, and I can just summarize them both real quick.
Undefined variables are ones with no assigned variable, while null will make variables have no variable, or be empty. They are very similar, but I think the best way to explain it is, variables with a null value are purposely programmed to have no value, while undefined variables were never given a value.
Operators
The next section was operators. There are many types of operators.
Assignment Operators
The following are assignment operators. We will use variables x & y as examples.
Assigment Operator | Description |
---|---|
x = y | Values of y is stored in x |
x += y | x + y is stored in x. The same as x = x + y |
x -=y | x - y is stored in x. The same as x = x - y |
x *= y | x * y is stored in x. The same as x = x * y |
x /= y | x / y is stored in x. The same as x = x / y |
x %= y | x % y is stored in x. The same as x = x % y |
x **= y | x ** y is stored in x. The same as x = x ** y |
Arithmetic Operators
Arithmetic operators, even if you are not a programmer, are probably easy to recognize.
- Addition (+)
- Subbtraction (-)
- Multiplication (*)
- Division (/)
- Modulus (aka remainder) (%)
- Exponential (**)
Comparison Operators
Next we had the comparison operators, or operators used to compare values.
Assigment Operator | Description |
---|---|
x == y | Equal in value only |
x === y | Equal in value and data type. Note: Use this instead of == |
x != y | Not equal |
x > y | Greater than |
x < y | Less than |
x >= y | Greater than or equal to |
x <= y | Less than or equal to |
Logical Operators
Three of the most common logical operators are &&(when both conditions on each side are the same, returns true, else false), ||(when either condition is true, returns true, else false), and !(negates the value, true beceomes false, false becomes true).
Increment and Decrement Operators
Increment operators increase a value stored in a variable, while a decrement operator decreases the value. Both can be done pre or post incrementally, though most of the time, you would use a post increment.
let count = 0;
console.log(++count) //pre increment
console.log(count--) //post increment
Window Methods
JavaScript has a few methods for pop ups in the browser.
Window alert()
Alert() displays an alert box with a message and an OK button. It is usuually used for testing.
alert(put your message here)
Window prompt()
Prompt() displays a prompt box that takes an input from the browser to take input values and that data can be stores into a variable.
prompt('required text', 'optional text')
Window confirm()
The confirm method will display a box with a message, along with an OK and cancel button. This box is typically used to ask a user for perimission to execute something. Clicking OK will return a true value, and Cancel a false value.
confirm('message here')
Date Object
Now, let's talk about how we can grab current time using JavaScript Date Object. Here is how to create a Date Object and its methods:
const now = new Date() //How to create Date object
//we are going to use a variable called time to contain set equal to method
time = now.getFullYear() //gets the year
time = now.getMonth() //gets month, will be from 0-11
time = now.getDate() //gets the date of the month (1-31)
time = now.getDay() //gets number of day of the week (0-6, Sunday-Saturday)
time = now.getHours() //gets current hour
time = now.getMinutes() //gets current minutes
time = now.getSeconds() //gets the current second
time = now.getTime() //gets the amount of seconds past since January 1, 1970
To print value for readibility, you may have to manipulate them. For example, for both getMonth() and getDay(), you have to add 1, as their index starts at 0.
And this is the end of my recap of Day 3 of 30DaysofJavaScript. My favorite part was learning about the Date Object, as time methods will be very useful to have for projects in the future.
Day 4 should be coming out soon! If you want to know when it comes out, subscribe to the blog below! Share if you found my content interesting, and consider buying me some boba with the link down below.
Until next time, wherever, and whenever you are, have an amazing day! Signing off.
If you liked this and would like to support, click the link below to send me a boba!!! 🧋